In App development, we always encounter the situation of using WebView, for example, we open webpage A, and then click the link in A to jump to B. If at this time, we press the return key of the system, the expected return is to return A, instead of pushing to a Native page.
But the truth is, if you don’t do special treatment, then it’s very likely not the expected effect (B -> A). However, we only need to modify the code to solve it.
Again, to be clear, our expectations
- If the webview has a history that can be rolled back, when the system back button is clicked, the webview history rolls back
- Otherwise, perform system rollback and return to the previous native interface
core code used
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 twenty one twenty two
|
WebViewController ? _webviewController ; WillPopScope ( onWillPop: () => _exitApp ( context ), child: xxx , } Future < bool > _exitApp ( BuildContext context ) async { if ( await _webviewController ! . canGoBack ()) { print ( "onwill goback" ); _webviewController ! . goBack (); return Future . value ( false ); } else { debugPrint ( "_exit will not go back" ); return Future . value ( true ); } }
|
- The WebViewController instance controllerGlobal is used to judge the detection and perform webview history rollback.
- Use WillPopScope to monitor the system’s return key call, and perform system return or rollback WebView history
- Here, controllerGlobal!.canGoBack is used to determine whether the webview history can be rolled back
- If you need to perform webview rollback history, call controllerGlobal!.goBack(), otherwise respond to system rollback
complete example code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 twenty one twenty two twenty three twenty four 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
|
import 'dart:async' ; import 'package:flutter/material.dart' ; import 'package:webview_flutter/webview_flutter.dart' ; void main () => runApp ( MaterialApp ( home: WebViewExample ())); class WebViewExample extends StatefulWidget { @ override _WebViewExampleState createState () => _WebViewExampleState (); } class _WebViewExampleState extends State < WebViewExample > { WebViewController ? _webviewController ; Future < bool > _exitApp ( BuildContext context ) async { if ( await _webviewController ! . canGoBack ()) { print ( "onwill goback" ); _webviewController ! . goBack (); return Future . value ( false ); } else { debugPrint ( "_exit will not go back" ); return Future . value ( true ); } } @ override Widget build ( BuildContext context ) { return WillPopScope ( onWillPop: () => _exitApp ( context ), child: Scaffold ( appBar: AppBar ( title: const Text ( 'Flutter WebView example' ), // This drop down menu demonstrates that Flutter widgets can be shown over the web view. ), // We're using a Builder here so we have a context that is below the Scaffold // to allow calling Scaffold.of(context) so we can show a snackbar. body: Builder ( builder: ( BuildContext context ) { return WebView ( initialUrl: 'http://droidyue.com' , javascriptMode: JavascriptMode . unrestricted , onWebViewCreated: ( WebViewController webViewController ) { _webviewController = webViewController ; }, ); }), ), ); } }
|
After using the above code, you can easily implement the priority response WebView history fallback.
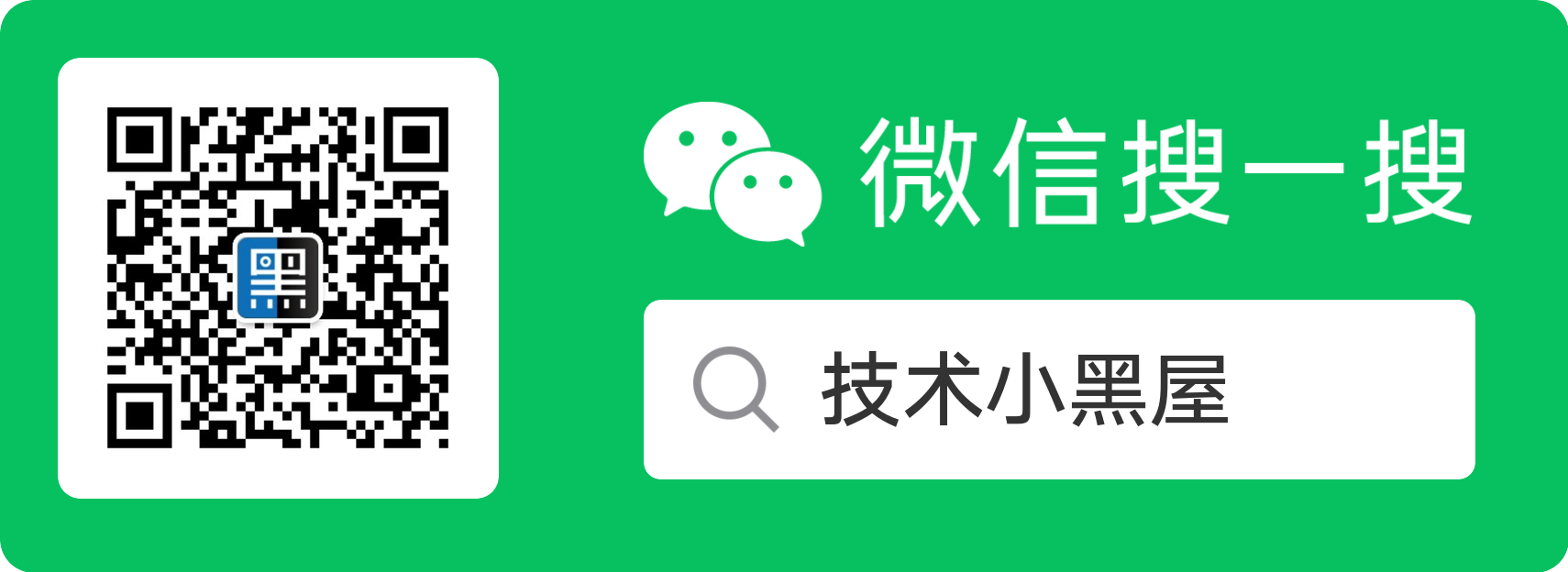
This article is reprinted from https://droidyue.com/blog/2022/07/12/flutter-webview-handle-self-goback-first/
This site is for inclusion only, and the copyright belongs to the original author.